mirror of
https://github.com/LuiCat/ArduinoTaikoController.git
synced 2025-01-18 15:54:08 +01:00
Fixed some problems in setup instructions and folder structure
This commit is contained in:
parent
9337f204c9
commit
fcf3612c05
37
README.md
37
README.md
@ -4,9 +4,13 @@ Sketch for Arduino based taiko game controller circuit
|
||||
|
||||
## Software Setup
|
||||
|
||||
### Arduino IDE
|
||||
|
||||
Install the latest version of Arduino IDE from the official website: [https://www.arduino.cc/en/Main/Software](https://www.arduino.cc/en/Main/Software)
|
||||
|
||||
To enable nintendo switch functionality, replace the following files with the ones provided in "setup" folder:
|
||||
### Modifications to Arduino IDE
|
||||
|
||||
Before starting Arduino IDE, to enable nintendo switch functionality, replace the following files with the ones provided in "setup" folder:
|
||||
|
||||
- <your arduino installation path>/hardware/arduino/avr/libraries/HID/src/HID.h
|
||||
- <your arduino installation path>/hardware/arduino/avr/libraries/HID/src/HID.cpp
|
||||
@ -15,15 +19,40 @@ Then copy the text in board.txt in "setup" folder and append it to the following
|
||||
|
||||
- <your arduino installation path>/hardware/arduino/avr/boards.txt
|
||||
|
||||
If you've successfully done all the modifications above, you should be able to see the board called "Nintendo Switch Controller" next time you start Arduino IDE:
|
||||
|
||||
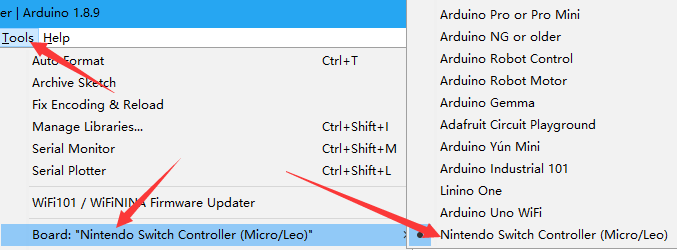
|
||||
|
||||
Please select this board before uploading the code as this is essential for your Arduino Leonardo to be recognized by Nintendo Switch.
|
||||
|
||||
### Keyboard or Nintendo Switch Controller
|
||||
|
||||
To enable or disable keyboard and Nintendo Switch controller functionality, remove or add two charactors "//" before these two lines in taiko_controller.ino:
|
||||
|
||||
- To enable Switch controller only
|
||||
```
|
||||
//#define ENABLE_KEYBOARD
|
||||
#define ENABLE_NS_JOYSTICK
|
||||
```
|
||||
- To enable keyboard only
|
||||
```
|
||||
#define ENABLE_KEYBOARD
|
||||
//#define ENABLE_NS_JOYSTICK
|
||||
```
|
||||
- To enable both (not tested)
|
||||
```
|
||||
#define ENABLE_KEYBOARD
|
||||
#define ENABLE_NS_JOYSTICK
|
||||
```
|
||||
|
||||
## Circuit Setup
|
||||
|
||||
### Materials
|
||||
|
||||
To setup the circuit, you need an Arduino Leonardo, a set of four piezo sensors, and four 1MΩ resistors for some special cases.
|
||||
|
||||
### Connect the Circuit
|
||||
|
||||
Connect the sensors to the 3.3v pin and the analog pins according to the diagram below:
|
||||
|
||||
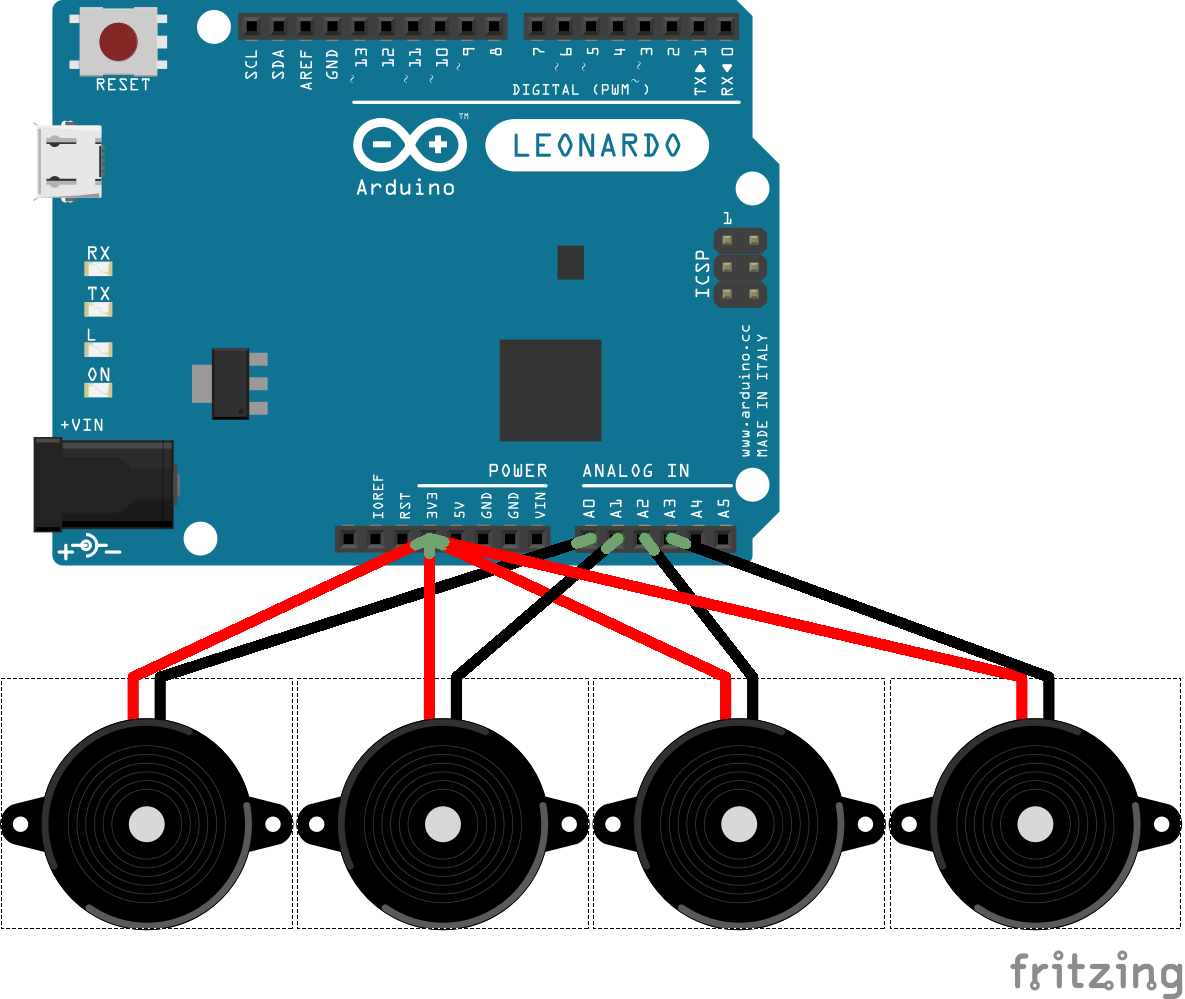
|
||||
@ -42,7 +71,9 @@ If the controller seems to be generating random inputs, you can fix this by plug
|
||||
|
||||
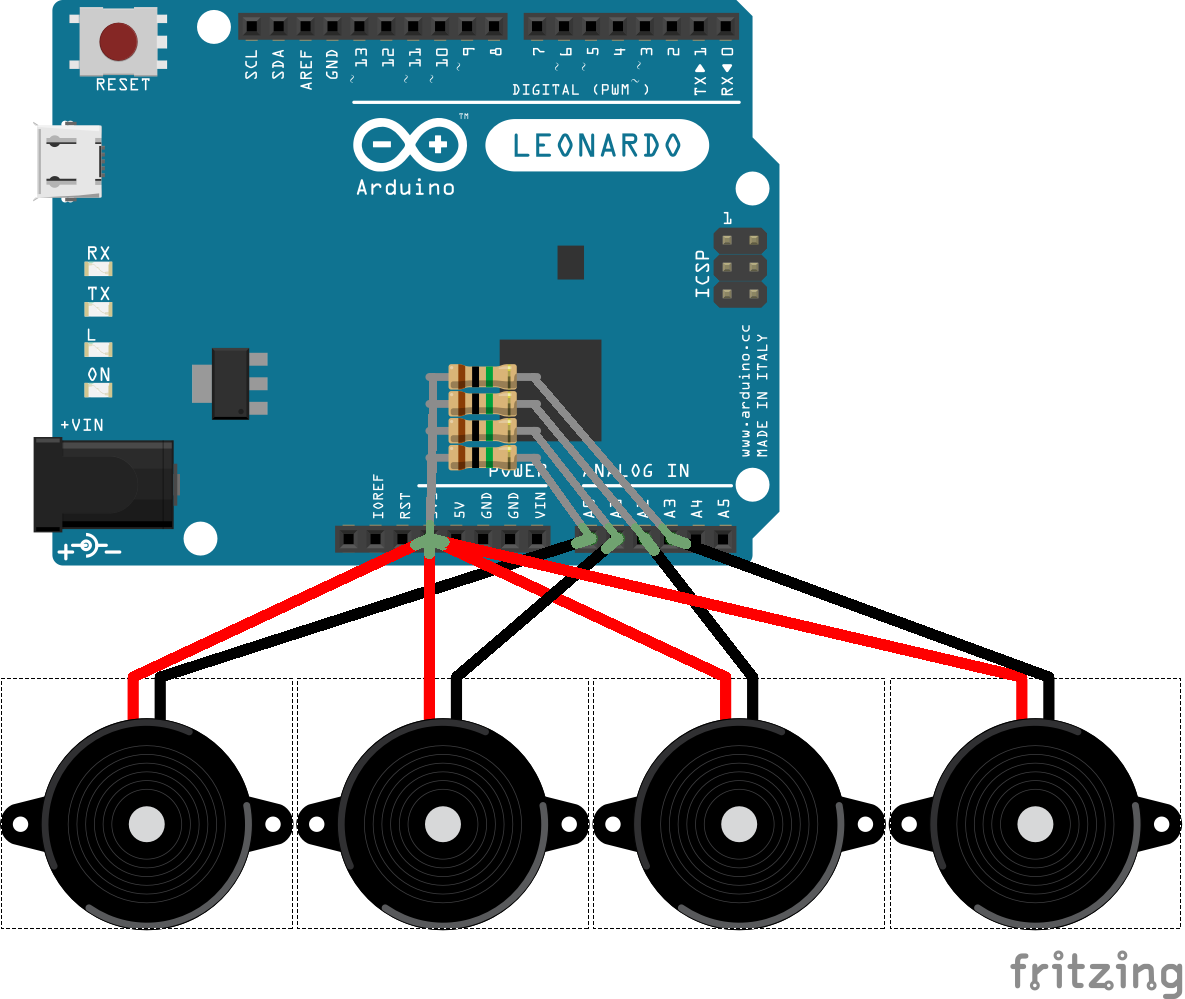
|
||||
|
||||
For best performance, the sensors must be piezo sensors (a.k.a. peizo speakers, contact microphones). No guarantee if other types of sensors will simply work, but if analog signals with voltage ranged 0-5V are fed into analog pins, this setup should be good to go.
|
||||
### Notes
|
||||
|
||||
For best performance, the sensors must be piezo sensors (a.k.a. piezo speakers, contact microphones). No guarantee if other types of sensors will simply work, but if analog signals with voltage ranged 0-5V are fed into analog pins, this setup should be good to go.
|
||||
|
||||
For further improvements, you can use some diodes to limit the voltage of the piezo sensors, or use a 2.5v power supply, but this won't matter in most cases, at least on my side.
|
||||
|
||||
@ -60,6 +91,8 @@ To deal with four analog inputs, we read the sensor levels one at a time, and on
|
||||
|
||||
To deal with Nintendo Switch, I used the HID descriptor for Hori's Pokken fightstick to let Switch trust Arduino as a valid controller device (see the [credits](#credits) section). The default buttons from the four sensors are the analog stick buttons (press the sticks down) and the trigger buttons (ZL and ZR).
|
||||
|
||||
As VID and PID of the controller have to be the specific value, the setup to boards.txt is essential. Also, Switch seems also to be judging the device strictly by the first-come HID descriptor of the device, so Arduino's default HID behavior have to be altered to have our customized HID descriptor to work.
|
||||
|
||||
## Parameters (with suggested values)
|
||||
|
||||
#### min_threshold = 15
|
||||
|
@ -86,6 +86,13 @@ void HID_::AppendDescriptor(HIDSubDescriptor *node)
|
||||
descriptorSize += node->length;
|
||||
}
|
||||
|
||||
void HID_::PrependDescriptor(HIDSubDescriptor *node)
|
||||
{
|
||||
node->next = rootNode;
|
||||
rootNode = node;
|
||||
descriptorSize += node->length;
|
||||
}
|
||||
|
||||
int HID_::SendReport(uint8_t id, const void* data, int len)
|
||||
{
|
||||
auto ret = USB_Send(pluggedEndpoint, &id, 1);
|
||||
@ -95,6 +102,11 @@ int HID_::SendReport(uint8_t id, const void* data, int len)
|
||||
return ret + ret2;
|
||||
}
|
||||
|
||||
int HID_::SendRaw(const void* data, int len)
|
||||
{
|
||||
return USB_Send(pluggedEndpoint | TRANSFER_RELEASE, data, len);
|
||||
}
|
||||
|
||||
bool HID_::setup(USBSetup& setup)
|
||||
{
|
||||
if (pluggedInterface != setup.wIndex) {
|
||||
|
@ -3,7 +3,7 @@
|
||||
# Nintendo Switch Controller for Micro/Leonardo #
|
||||
##############################################################
|
||||
|
||||
ns_con.name=Nintendo Switch Controller (Micro)
|
||||
ns_con.name=Nintendo Switch Controller (Micro/Leo)
|
||||
|
||||
ns_con.vid.1=0x0F0D
|
||||
ns_con.pid.1=0x0092
|
||||
|
@ -8,22 +8,6 @@
|
||||
* https://github.com/progmem/Switch-Fightstick/blob/master/Joystick.h
|
||||
***/
|
||||
|
||||
// Functions added to HID_ class
|
||||
// Don't forget to add definitions in HID.h, which is located at:
|
||||
// <arduino installation path>\hardware\arduino\avr\libraries\HID\src\
|
||||
|
||||
void HID_::PrependDescriptor(HIDSubDescriptor *node)
|
||||
{
|
||||
node->next = rootNode;
|
||||
rootNode = node;
|
||||
descriptorSize += node->length;
|
||||
}
|
||||
|
||||
int HID_::SendRaw(const void* data, int len)
|
||||
{
|
||||
return USB_Send(pluggedEndpoint | TRANSFER_RELEASE, data, len);
|
||||
}
|
||||
|
||||
/***
|
||||
* Descriptor modified from
|
||||
* progmem/Switch-Fightstick/Joystick.c
|
||||
@ -100,4 +84,3 @@ void Joystick_::sendState()
|
||||
}
|
||||
|
||||
Joystick_ Joystick;
|
||||
|
Loading…
x
Reference in New Issue
Block a user